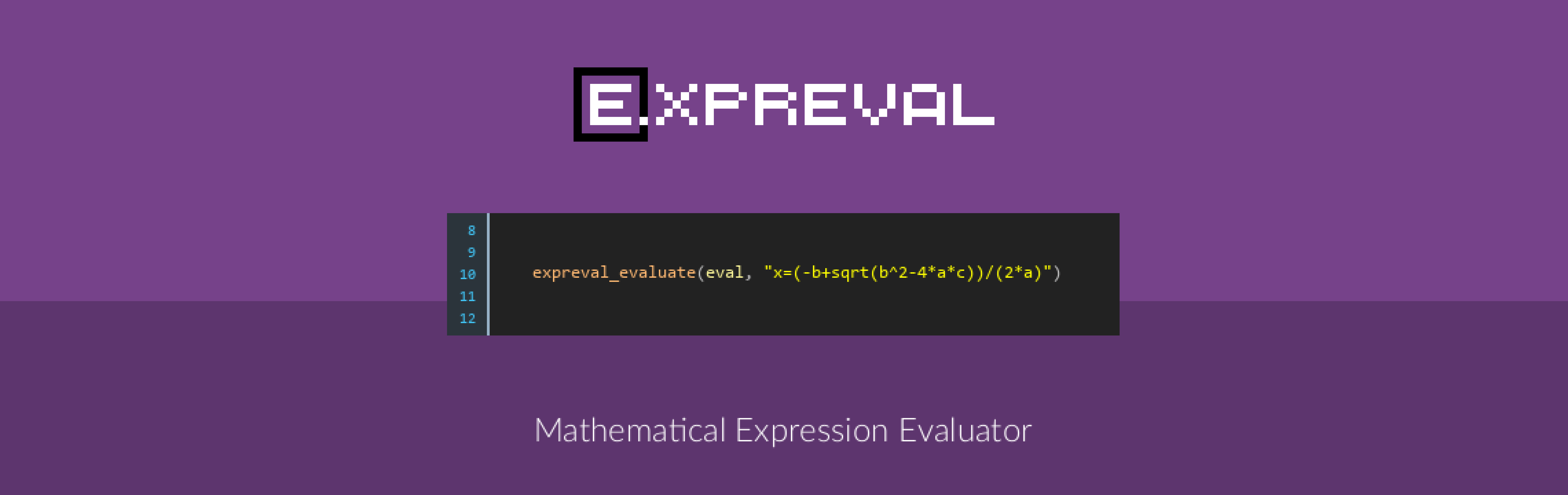
Expression Evaluator for GameMaker
A downloadable extension
Available on YoYo Games marketplace
This extension provides a mathematical expression evaluator. Mathematical expressions can be passed to it as a string, and solved. This allows mathematical expressions to be stored as strings, and be generated or altered at run-time.
Basic Usage
One-shot calculation
In the most basic usage, variables are loaded into the a new expreval, and an expression is evaluated. The output variables can then be read.
// create expression var eval = expreval_create(); // set up variables expreval_write_variable(eval, "a", 123); // evaluate expression (intermediate variables are created automatically expreval_evaluate(eval, "b=2*a;c=sin(b)"); // grab output variables var retval = expreval_read_variable(eval, "c"); show_debug_message("Returned value: " + string(retval)); expreval_destroy(eval);
Multiple expressions can be evaluated, by separating them with a semicolon in the expression string.
Any temporary or output variables used in the expression will be automatically created, and made available for reading. Referencing an undefined variable will cause an error.
exprevals must be destroyed after they are no longer used to avoid a memory leak.
Re-running an expression
expreval can load an expression to be run later by using the expreval_load() and expreval_run() functions instead of expreval_evaluate(). This allows
// create expression var eval = expreval_create(); // load expression (this loads it but does not run it yet expreval_load(eval, "x=(-b+sqrt(b^2-4*a*c))/(2*a)"); // time expreval for(var i=0; i<10; i++) { expreval_write_variable(eval, "a", 1.0); expreval_write_variable(eval, "b", i*2); expreval_write_variable(eval, "c", i); expreval_run(eval); var retval = expreval_read_variable(eval, "x"); show_debug_message("Returned value: " + string(retval)); } expreval_destroy(eval);
Advanced usage
expreval_create()
Creates a new expreval object in the extension. This returns a numerical index which is used in other functions. The expreval object will remain available for the duration of the game or until expreval_destroy() is called. This allows the expression to be modified and evaluated multiple times without having to be recreated from scratch each time
expreval_destroy(index)
Unloads the expreval object inside the extension. Use this when the file is no longer needed to avoid memory leaks
expreval_exists(index)
Returns true or false depending on whether the provided index exists inside the extension or not.
expreval_write_variable(index, varname, value)
Adds or modifies the value of a variable inside an expression. This can be called multiple times to update the variable.
expreval_read_variable(index, varname)
Returns the value of the variable. This is used after evaluating an expression to read the output value. If the variable does not exist, 0 is returned.
expreval_evaluate(index, expression)
Loads and evaluates a mathematical expression. Returns 1 if successful, returns 0 if unsuccessful. Will also output error messages in the console.
expreval_load(index, expression)
Loads a mathematical expression, but does not evaluate it immediately until exrpeval_run() is called
expreval_run(index)
Runs the previously loaded expression. Can be run after either expreval_load() or expreval_evaluate(). Returns 1 if successful, returns 0 if unsuccessful. Will also output error messages in the console.
expreval_set_debug_mode(mode)
Expreval will output things in the console. This sets the verbosity of expreval. The following values are possible:
- EXPREVAL_DEBUG_NONE: nothing will be outputted
- EXPREVAL_DEBUG_ERROR: only errors will output
- EXPREVAL_DEBUG_WARNING: warnings and errors (this is the default value)
- EXPREVAL_DEBUG_INFO: info, warnings, and errors
- EXPREVAL_DEBUG_DEBUG: everything
Available expressions
abs(x) - Absolute value of x
mod(x, y) - modulo x of y (i.e. remainder of x when divided by y)
ipart(x) - the integer part of x
fpart(x) - the fractional part of x
min(x, y, z ...) - returns the smallest value of all the parameters
max(x, y, z ...) - returns the largest value of all the parameters
sqrt(x) - square root of x
poly(x, ...) - calculates a polynomial, the arguments are coefficients. E.g.: poly(x, 2, 3, 4, 5) becomes 2x^3 + 3x^2 + 4x + 5
Rounding
ceil(x) - round x to the nearest integer towards positive infinity
floor(x) - round x to the nearest integer towards negative infinity
clip(x, min, max) - returns x clipped to min and max (this is equivalent to GM's clamp() function)
clamp(x, min, max) - wraps x between min and max (note: this is not the same as GM's clamp() function, see above)
rescale(p, o1, o2, n1, n2) - rescales p as a fraction of range o1 and o2 to the range n1 and n2 (this is similar to GM's lerp() function but extends the lerp fraction)
Trigonometry
sin(x), cos(x), tan(x) - trigonometric functions, in radian
asin(x), acos(x), atan(x) - inverse trigonometric functions, in radians
atan(x, y) - quadrant-aware inverse tangent function (equivalent to atan2() function in GM and other languages)
sinh(x), cosh(x), tanh(x) - hyperbolic trigonometric functions, in radians
deg(x) - converts x from radians to degrees
rad(x) - converts x from degrees to radians
rect2pol(x, y, &distance, &angle) - converts rectangular coordinates x and y to polar coordinates distance and angle. This is similar to using point_distance() and point_direction() in GM
pol2rect(distance, angle, &x, &y) - converts polar coordinates distance and angle to rectangular coordinates x and y. This is similar to using lengthdir_x() and lengthdir_y() in GM.
Logarithms
log(x) - base 10 logarithm of x
ln(x) - base e logarithm of x
exp(x) - e raised to the power of x
logn(x, y) - base y logarithm of x
Random numbers
rand(&seed) - random number between 0 and 1, also reads and updates seed variable (seed can be written to directly to provide a starting seed)
random(min, max, &seed) - random number between min and max, reads and updates seed variable
randomize(&seed) - randomizes the seed used
Conditionals and Boolean
if(condition, true_exp, false_exp) - provides an if statement. If condition is non-zero, then true_exp is evaluated and returned, else false_exp is evaluated and returned
select(condition, neg_exp, zero_exp, pos_exp) - selects between neg_exp, zero_exp, pos_exp depending on whether condition is negative, zero, or positive
select(condition, neg_exp, zeropos_exp) - selects between neg_exp, zeropos_exp depending on whether condition is negative, or zero or positive.
equal(x, y) - returns 1 if x and y are equal, returns 0 if not (equivalent to x == y)
above(x, y) - returns 1 if x is greater than y (equivalent to x > y)
below(x, y) - returns 1 if x is less than y (equivalent to x < y)
and(x, y) - returns 1 if x and y evaluate to true (equivalent to x && y)
or(x, y) - returns 1 if x or y evaluate to true (equivalent to x || y)
not(x) - returns 1 if x evaluates to false (equivalent to !x)
Constants
PI - value of pi: 3.14159265358979323846
E - value of natural number e: 2.7182818284590452354
Known issues
- expreval is about 50 times slower than VM native calculations, and probably several times that versus YYC
Status | Released |
Category | Assets |
Rating | Rated 4.0 out of 5 stars (1 total ratings) |
Author | meseta |
Made with | GameMaker |
Tags | GameMaker |
Code license | MIT License |
Links | YoYo Marketplace |
Download
Click download now to get access to the following files: